WTF Solidity极简入门: 17. 库合约 站在巨人的肩膀上
我最近在重新学 Solidity,巩固一下细节,也写一个“WTF Solidity极简入门”,供小白们使用(编程大佬可以另找教程),每周更新 1-3 讲。
所有代码和教程开源在 github: github.com/AmazingAng/WTF-Solidity
这一讲,我们用ERC721
的引用的库合约Strings
为例介绍Solidity
中的库合约(Library
),并总结了常用的库合约。
库合约
库合约是一种特殊的合约,为了提升Solidity
代码的复用性和减少gas
而存在。库合约是一系列的函数合集,由大神或者项目方创作,咱们站在巨人的肩膀上,会用就行了。
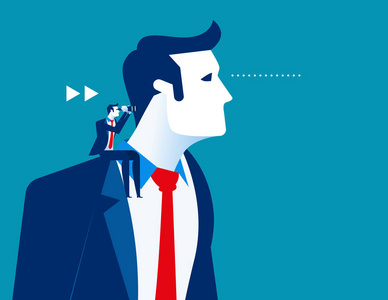
他和普通合约主要有以下几点不同:
- 不能存在状态变量
- 不能够继承或被继承
- 不能接收以太币
- 不可以被销毁
需要注意的是,库合约重的函数可见性如果被设置为public
或者external
,则在调用函数时会触发一次delegatecall
。而如果被设置为internal
,则不会引起。对于设置为private
可见性的函数来说,其仅能在库合约中可见,在其他合约中不可用。
Strings库合约
Strings库合约
是将uint256
类型转换为相应的string
类型的代码库,样例代码如下:
library Strings {
bytes16 private constant _HEX_SYMBOLS = "0123456789abcdef";
/**
* @dev Converts a `uint256` to its ASCII `string` decimal representation.
*/
function toString(uint256 value) public pure returns (string memory) {
// Inspired by OraclizeAPI's implementation - MIT licence
// https://github.com/oraclize/ethereum-api/blob/b42146b063c7d6ee1358846c198246239e9360e8/oraclizeAPI_0.4.25.sol
if (value == 0) {
return "0";
}
uint256 temp = value;
uint256 digits;
while (temp != 0) {
digits++;
temp /= 10;
}
bytes memory buffer = new bytes(digits);
while (value != 0) {
digits -= 1;
buffer[digits] = bytes1(uint8(48 + uint256(value % 10)));
value /= 10;
}
return string(buffer);
}
/**
* @dev Converts a `uint256` to its ASCII `string` hexadecimal representation.
*/
function toHexString(uint256 value) public pure returns (string memory) {
if (value == 0) {
return "0x00";
}
uint256 temp = value;
uint256 length = 0;
while (temp != 0) {
length++;
temp >>= 8;
}
return toHexString(value, length);
}
/**
* @dev Converts a `uint256` to its ASCII `string` hexadecimal representation with fixed length.
*/
function toHexString(uint256 value, uint256 length) public pure returns (string memory) {
bytes memory buffer = new bytes(2 * length + 2);
buffer[0] = "0";
buffer[1] = "x";
for (uint256 i = 2 * length + 1; i > 1; --i) {
buffer[i] = _HEX_SYMBOLS[value & 0xf];
value >>= 4;
}
require(value == 0, "Strings: hex length insufficient");
return string(buffer);
}
}
他主要包含两个函数,toString()
将uint256
转为string
,toHexString()
将uint256
转换为16进制
,在转换为string
。
如何使用库合约
我们用Strings
库合约的toHexString()
来演示两种使用库合约中函数的办法。
利用using for指令
指令
using A for B;
可用于附加库合约(从库 A)到任何类型(B)。添加完指令后,库A
中的函数会自动添加为B
类型变量的成员,可以直接调用。注意:在调用的时候,这个变量会被当作第一个参数传递给函数:// 利用using for指令
using Strings for uint256;
function getString1(uint256 _number) public pure returns(string memory){
// 库合约中的函数会自动添加为uint256型变量的成员
return _number.toHexString();
}通过库合约名称调用函数
// 直接通过库合约名调用
function getString2(uint256 _number) public pure returns(string memory){
return Strings.toHexString(_number);
}
我们部署合约并输入170
测试一下,两种方法均能返回正确的16进制string
“0xaa”。证明我们调用库合约成功!
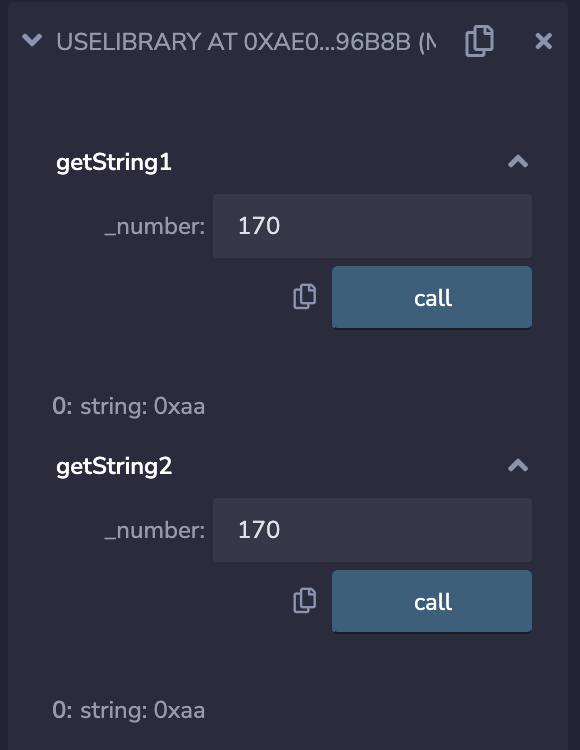
总结
这一讲,我们用ERC721
的引用的库合约Strings
为例介绍Solidity
中的库合约(Library
)。99%的开发者都不需要自己去写库合约,会用大神写的就可以了。我们只需要知道什么情况该用什么库合约。常用的有: